The code removes the element at index 3. This is why we explicitly check if a matching object was found before removing Start Your Free Software Development Course, Web development, programming languages, Software testing & others. int[] arr = new int[]{1, 2, 3, 4}; arr = null; This will clear out the array. Comment * document.getElementById("comment").setAttribute("id","a465525eb60466eb0cf2b6841543a426");document.getElementById("fce79cd32f").setAttribute("id","comment"); Save my name, email, and website in this browser for the next time I comment. However, deleting elements from an array can be a challenging task, as the size of the array is fixed once we initialize it. Finally, we return the list back to an integer array using again the ToArray() method. C# Program to remove the elements which occurred more than If it is not present, we can add the same using the push method. Improving the copy in the close modal and post notices - 2023 edition. Do pilots practice stalls regularly outside training for new certificates or ratings? Compare the value one by one in a for loop, How to remove an element from an object array, How to remove multiple elements from an object array, Add remove methods to Array interface (prototype), Simple Performance comparison indexOf loop vs for loop, How to check if an object implements an interface in Typescript, Typescript enum get key by value, value by key, use as object key, TypeScript How to set a default value with Interface, How to generate Number Range Array in TypeScript, How To Remove Items From Python List | Python Tutorial | Python List Remove Method. If playback doesnt begin shortly, try restarting your device. You won't notice the difference, really.If you move to .NET 2.0, instead, you could use a List instead of the ArrayList. We can achieve the same results for deleting elements from an array by using the RemoveAll() method: First, we create a new List() object from the inputArray array argument. shift Removes from the beginning of an Array. Inside the reduce method, we can use the indexOf method to check if the current element is already present in the accumulator array. Is renormalization different to just ignoring infinite expressions? How can I add new array elements at the beginning of an array in JavaScript? When the current element meets the criterion, copy it into the next available space in the array; when it doesn't skip it. The function we passed to the Data Structure & Algorithm-Self Paced(C++/JAVA) Data Structures & Algorithms in Python; Explore More Self-Paced Courses; Programming Languages. This can be attributed to the fact that LINQs Where() extension method internally uses deferred execution and optimizes the code by generating code that is closer to the CPU instructions. It allows for efficient insertion and removal of elements at any position in the list, which makes it useful in situations where elements need to be dynamically added or removed. In a certain operation I want to remove all elements of this synchronized list and process them. In the above program, we input 8 elements for an array arr[] from the user. I feel like I'm pursuing academia only because I want to avoid industry - how would I know I if I'm doing so? To download the source code for this article, you can visit our, How to Create a Custom Authorize Attribute in ASP.NET Core, Vendor-Agnostic Telemetry Using OpenTelemetry Collector in .NET. If the pos is greater than the num+1, the deletion of the element is not possible and jump to step 7. I can also use framework 2.0 but in either case, will be using an arraylist. WebBelow are the techniques used to remove the duplicate elements as follows. To remove a specific object from an element in swift, we can use multiple ways of doing it. WebVisual Representation. 1. To remove all the occurrences of an element in an array, we will use the following 2 approaches: Using Methods; Without using methods or With Conditional // Remove all elements that are less than 3. auto new_end = std::remove_if(vec. Space complexity: O(1n) = O(n), [best approach if you want to leave the original array as-is], For n elements in the array: Array elements after removing prime numbers: C program to calculate median of an array, C program to check prime numbers in an array, Initialising byte array with decimal, octal and hexadecimal numbers in C, C program to swap first element with last, second to second last and so on (reversing elements), C program to find nearest lesser and greater element in an array, C program to merge two arrays in third array which is creating dynamically, C program to create array with reverse elements of one dimensional array, C program to count total number of elements divisible by a specific number in an array, C program to create a new array from a given array with the elements divisible by a specific number, C program to find second largest elements in a one dimensional array, C program to find two largest elements in a one dimensional array, C program to find second smallest element in a one dimensional array, C program to find two smallest elements in a one dimensional array, C Program to Cyclically Permute the Elements of an Array, C program to accept Sorted Array and do Search using Binary Search, C Program to find the Biggest Number in an Array of Numbers using Recursion, C program to print the number of subset whose elements have difference 0 or 1, C program to read and print One Dimensional Array of integer elements, C program to calculate Sum, Product of all elements, C program to find Smallest and Largest elements from One Dimensional Array Elements, C program to replace all EVEN elements by 0 and Odd by 1 in One Dimensional Array, C program to merge Two One Dimensional Arrays elements, C program to Add and Subtract of Two One Dimensional Array elements, C program to find a number from array elements, C program to sort array elements in ascending order, C program to swap adjacent elements of a one dimensional array, C program to find occurrence of an element in one dimensional array, C program to sort an one dimensional array in ascending order, C program to sort an one dimensional array in descending order, C program to delete given element from one dimensional array, C program to find the first repeated element in an array, C program to calculate the sum of array elements using pointers as an argument, C program to find the sum of the largest contiguous subarray, C program to split an array and add the first half after the second half of the array, C program to generate pascal triangle using the array, C program to access array element out of bounds, C program to print alternate elements of the array, C program to print the non-repeated elements of an array, C program to find the total of non-repeated elements of an array, C program to find the missing number in the array, C program to find the missing number in the array using the bitwise XOR operator, C program to segregate 1's and 0's in the array, C program to find the difference between the largest and smallest element in the array, C program to print the square of array elements, C program to find two elements whose sum is closest to zero, C program to check a given number appears more than N/2 times in a sorted array of N integers, C program to find the median of two sorted arrays with same using simple merge-based O(n) solution, C program to find the median of two arrays using a divide and conquer-based efficient solution, C program to find the intersection of two arrays, C program to find the union of two arrays, C program to find the size of the array using macro, C program to find the ceiling element of the given number in the sorted array, C program to find the floor element of the given number in the sorted array, C program to create an integer array and store the EVEN and ODD elements in a different array, Generally Accepted Accounting Principles MCQs, Marginal Costing and Absorption Costing MCQs, To check prime numbers, we declare a function, Then, we are deleting the prime numbers, by checking elements using condition. The second argument is the number of elements that will be deleted. This method call removes two elements. Run an inner loop from i + 1 to size. In the above example mentioned, we have iterated every element of the ar array by using the method of foreach. splice method instead. First, lets create an array of String. If it does not exist, we return the inputArray array as is, as there are no elements to remove. For the first indexOf call, it doesnt find the element even though the values are the same as the first element. If the elementToRemove is not found in the inputArray, we simply return the inputArray. If you are going to be doing a lot of deleting in a large list, though, consider using a linked list. Typically, you keep the length in a separate variable. Use the splice() method to remove elements from an array, e.g. In >&N, why is N treated as file descriptor instead as file name (as the manual seems to say)?
Array.findIndex method gets However, we need to be cautious when we use arrays by reference. However the code I've written does not work. However, the other two elements were added by push(obj) method. curl --insecure option) expose client to MITM.
Join our 20k+ community of experts and learn about our Top 16 Web API Best Practices. Here, we have 7 elements in an array, and the user wants to delete the 8th position element, which is impossible. C programs If you need to remove multiple elements from an object array, you need to remove them in a for-loop. Inside the main () function, initialize the sorted array. at the provided index). Step3: At last, we need to compare the last element with its preceding element. Step 1: Input the size of the array arr[] using num, and then declare the pos variable to Relates to going into another country in defense of one's people. Correct. Arrays are the fundamental data structure in programming, and its common to encounter situations where we need to remove duplicates from them. Then, we decrease the array size by one, with the use of the Array.Resize() method. Programming stuffs with full example code which you can download and try easily. If it starts from the end, it doesnt affect the result. Check out, 10 Things You Should Avoid in Your ASP.NET Core Controllers. The resultant array consists of unique array. The remaining elements have been moved to the left and the new array has a size of five elements.
You could construct a Set from the array and then create an array from the set. I guess for-loop is used in indexOf to find the index. here is a pseudocode that you can work with: If you want to delete easily and efficiently without using loop you can use memcpy. Would spinning bush planes' tundra tires in flight be useful? In a certain operation I want to remove all elements of this synchronized list and process them. We can use the indexOf method with a combination of other methods to remove the duplicates from an array. The value is the same but the object itself is different. Finally, we return the concatenation of these two segments using the Concat() method and convert the resulting IEnumerable to an array using the ToArray() method.
In this article, we looked at six different methods that allow us to delete elements from an array. rev2023.4.5.43379. Using the forEach method alone, we cannot remove duplicate elements from the array in javascript. How can I remove a specific item from an array in JavaScript? If the function returns a truthy value, the array element gets added to the Connect and share knowledge within a single location that is structured and easy to search. 3 How do you remove an element from an array? This method requires the creation of a new array. First I would not call the function delete_cards as it suggests that it deletes multiple cards which it does not - just delete_card would make The filter method does not change the contents of the original array, instead check or uncheck the columns where you want to remove the duplicates.
This is the only way that ensures that when you remove an element your counting isn't messed up. After completion of the loop, the un array contains only unique elements from the original array. Then, we create a second one from the right next position of the calculated index to the end of the inputArray. The example below shows removing duplicate elements from the array using the indexOf method in javascript as follows. Submitted by IncludeHelp, on March 09, 2018. JavaTpoint offers too many high quality services. { Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. Could my planet be habitable (Or partially habitable) by humans? How can I remove a specific item from an array in JavaScript? In this article, well explore and learn the different methods for removing duplicates from arrays in JavaScript: using the filter() method, the Set() method, the forEach() method, Reduce() method, and Index () method. We can use the foreach method with the combination of the array method to remove the duplicates from the array. If you want to remove an element in the middle of the array, you need to first know the index of the target element. Array.splice The routine is run tens of times per second. Can I disengage and reengage in a surprise combat situation to retry for a better Initiative? The easiest approach if you're trying to filter certain values from an array, however, is to just copy to a new array. Shift all the elements from index + 1 (index 2 to 4) by 1 position to the left.
The content you requested has been removed. Use the filter() method to filter a TypeScript array condition. THE CERTIFICATION NAMES ARE THE TRADEMARKS OF THEIR RESPECTIVE OWNERS. ALL RIGHTS RESERVED. It takes an index and amount of items to delete starting from that index. Removing duplicate elements from an array in PHP. To remove the same, we have used the filter method on the array, and also we have passed the callback function. C one-dimensional array programs, In this C program, we are going to learn how can we check and delete prime numbers from an array? Use Array.Copy () To Delete an Element From an Array First, we will manually remove it with the ArrayCopy () extension method: public int[] DeleteWithArrayCopy(int[] inputArray, int elementToRemove) { var indexToRemove = Array.IndexOf(inputArray, elementToRemove); if (indexToRemove < 0) { return C Program to delete the duplicate elements in an array - Try to delete the same numbers present in an array. from array in JavaScript ? Therefore, only the two elements were removed here. The variability isn't going to matter, either it will range between 0 movements in a single cycle, to a worst case of about 50 movements. To remove duplicate elements below, we have used set, filter, reduce, for each, and IndexOf methods as follows.
Remove Array Elements With the Splice Function Using Splice is the most versatile way to remove an individual element from an array. Arrays do not have built-in deletion capabilities. How many sigops are in the invalid block 783426? Check this post if you want to remove duplicates.8 ways to remove duplicates from Array in TypeScriptThis page shows 8 ways to remove duplicates from an array. If you want to sort the array on your needs, you need to write your own sorting logic. Sometimes, an array may contain duplicate values, which can be problematic when processing or analyzing the data. it. Given an array of integer elements and we have to remove prime numbers using C program. Home It might cause a performance issue. The We can use the reduce method to remove duplicate elements from the array in javascript. And after two or more elements are removed. To learn more, see our tips on writing great answers. You should end up with two indexes, both starting at 0. Any idea would be greatly appreciated because I am truly stuck and I don't understand what I am doing wrong, (I still need to clean this answer up a bit more later--which I will when I make the time.). Why can I not self-reflect on my own writing critically? They are used to store multiple items but allow only the same type of data.Add/Update an array. The std::list container is a built-in container in C++ that provides a linked-list-based implementation. It removes elements one by one and shifts all other elements as an item is removed. 02-23-2015 09:40 PM. Removing an element from Array using for loop. You need to do it in a loop and use the shift register to maintain the array between Remove empty elements from an array in Javascript, How to insert an item into an array at a specific index (JavaScript), Sort array of objects by string property value. @user3386109, I still need to clean this answer up some more another day, and perhaps delete it and move it entirely to become an answer elsewhere, but I at least quickly added in the O(n) in-place solution to the beginning of my answer. I've also written a guide on After the completion of the loop, the un array will contain only unique elements. To remove from the end of the (big) index. The deletion of the element does not affect the size of an array. This page shows 8 ways to remove duplicates from an array. Dont confuse this with its similar cousin slice () that is used to extract a section of an array. When I built it with this tscondig, the difference becomes smaller for some reason Why? If you want to delete easily and efficiently without using loop you can use memcpy #include If you want to delete multiple elements, it needs to be done in a loop. If you often remove an element in many places, its better to define the function in the Array interface in the following way so that you can simply call the methods like array.remove(). It also covers the case where the element is object. I hadn't been decent programmer for a long time because I hadn't written unit test. Plagiarism flag and moderator tooling has launched to Stack Overflow! it returns a new array. In a reduced method, the array elements are combined and reduced into the final array based on the reducer function we passed. How to properly calculate USD income when paid in foreign currency like EUR? First I would not call the function delete_cards as it suggests that it deletes multiple cards which it does not - just delete_card would make things more clear. Full Stack Development with Following is the steps to remove a particular element from an array in C programming. In javascript, the array is a collection of values, including strings, objects, numbers, and other data types. rev2023.4.5.43379. WebFollowing is the steps to remove a particular element from an array in C programming. C# program to calculate the sum of array elements using the Linq Aggregate () method. Do pilots practice stalls regularly outside training for new certificates or ratings? If the target value is not found anymore, it breaks the loop. We will evaluate these methods to find the most efficient one in terms of speed, with thebenchmark class. In summary, removing duplicate elements from an array in javascript is a common task that every developer encounters at some point. So, here is a demo of that. Is there a connector for 0.1in pitch linear hole patterns? So, first, the user must define the position of the 8th element, which is the 4th, and then check whether the deletion is possible or not. C# program to calculate the total of employee's salaries using the Aggregate () method. Note: This solution requires that index is sorted (lowest first). Does disabling TLS server certificate verification (E.g. If you need to remove an object from a TypeScript array: The function we passed to the To subscribe to this RSS feed, copy and paste this URL into your RSS reader. In standard tuning, does guitar string 6 produce E3 or E2? Let's consider an example to demonstrate the deletion of the particular element using the user defined function in the C programming language. We achieve the same result as the previous methods, but with a more concise syntax, using LINQ. Step2: Now start for index 1 and compare with its adjacent elements (left and right elements) till the n-1 elements. The splice method changes the contents of the original array and returns an how to add elements to an array in TypeScript. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. Step 4: Compare the position of an element (pos) from the total no. WebBelow are the techniques used to remove the duplicate elements as follows. If we remove element 20 from the array, the execution will be, 1. We have logged output into the rd array. So, when we want to remove only one instance of an element from an array, the most efficient way is to use the ArraySegmentobject. Time complexity: best-case (nothing to filter out): O(n); worst-case (all elements must be filtered out 1-at-a-time): O(n^2) Is this even the right logic or i should try something completely different? First, we find the index position of the given element. We can remove the duplicate value from the array by adjusting the condition of an array. We hope that this EDUCBA information on Remove Duplicate Elements from Array Javascript was beneficial to you. I want to delete multiple elements from array using index array,this is my code: I expect print the player should be 1,2,6,7 instead of 1,2,3,4. It allows for efficient insertion and removal of elements at any position in the list, which makes it useful in situations where elements need to be dynamically added or removed. You can also assign a new array to that reference if you like: int[] arr = new int[]{1, 2, 3, 4}; arr = new int[]{6, 7, 8, 9}; How to remove multiple elements from array in JavaScript? Decent programmer should at least Json file is widely used for many purposes. Were sorry. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Step 3 Now, calls the duplicatesRemove () function and pass the array to it. replacing or adding new elements and returns an array containing the removed Please mail your requirement at [emailprotected] Duration: 1 week to 2 week. How do you remove an element from an array? It is ok to try-catch doesn't catch an error thrown in timer friend functions setTimeout, setInterval and setImmediate. get only the odd numbers from an array that contains both odd and even numbers. You could try and use delete array [index] This wont completely remove the element but rather sets the value to undefined. a) Play all cards first and then delete the cards played. How to solve this seemingly simple system of algebraic equations? So, it doesnt allocate new memory or copy any data, as we can verify from the last two columns, too. Instead, it checks whether the array still has the target value or not. We need to remove the element 20 (index 1) from the array. How do I remove multiple elements from an array in C++? is there any way to correct this? The in-place algorithm looks good, and like you said, it uses the same logic as the "copy" solution. Also, we can refactor this method to use the Array.Resize() method: Here, in the for loop, we shift one position to the left every element after the indexToRemove. How to "remove an element" from an array in C. You might also call this "filtering an array" in C. You can't resize statically-allocated arrays in C. Instead, you just Using filter () Method. 6 elements.Given your assumption that 5% of the elements will have to be moved, this means that you will move, on average, 6 * 12 * 0.05 elements per scan, i.e. Step 4 Further, the resultant updated array after removing the duplicates is printed using the fmt.Println () function. to 4. It will create a new array with unique values. We are declaring an array with some prime and non prime numbers and deleting prime numbers and then printing array elements with the numbers which are not prime. If there are multiple elements to delete, it calls it multiple times. This website or its third-party tools use cookies, which are necessary to its functioning and required to achieve the purposes illustrated in the cookie policy. You're going to need to think rather carefully about how to resolve this. WebStep1: Check for the first element if it is equal to or not equal to its succeeding element. By closing this banner, scrolling this page, clicking a link or continuing to browse otherwise, you agree to our Privacy Policy, Explore 1000+ varieties of Mock tests View more, By continuing above step, you agree to our, JavaScript Training Program (39 Courses, 24 Projects, 4 Quizzes), Angular JS Training Program (9 Courses, 7 Projects), Software Development Course - All in One Bundle. How to remove duplicate elements of an array in java? WebUse the splice () method to remove elements from an array, e.g. In this example, shi Array type does not have a built-in method for this task and we often resort to alternative techniques such as converting the Array to a List or utilizing LINQ to remove specific elements from the Array. Reduced into the final array based on the reducer function we passed (. Should Avoid in your ASP.NET Core Controllers method changes the contents of the ar array by adjusting the condition an. Remove them in a certain operation I want to sort the array in javascript the pos greater... Usd income when paid in foreign currency like EUR after completion of the ar array using! Download and try easily programming language in swift, we can not duplicate! Use framework 2.0 but in either case, will be, 1 in an array where we to! Indexof method in javascript is a common task that every developer encounters at some point written guide... Elements at the beginning of an array arr [ ] from the end of the index... Completely remove the element does not work to say ) improving the in. End of the inputArray this EDUCBA information on remove duplicate elements from the end of the element is found... Begin shortly, try restarting your device prime numbers using C program are the fundamental data structure in programming and... Odd numbers from an object array, and other data types written, well thought and well computer! You should Avoid in your ASP.NET Core Controllers encounter situations where we to. Returns an how to add elements to an integer array using the Linq Aggregate ( ) to! Swift, we find the most efficient one in terms of speed, with use! Filter ( ) method have used the filter ( ) method to remove them a! Value is the steps to remove want to remove duplicate elements from array. Requires that index and right elements ) till the n-1 elements technologists delete multiple elements from array c whether the array in?. Num+1, the un array contains only unique elements with a combination of other to! To delete, it doesnt find the index position of an array in java using.. In summary, removing duplicate elements of an element from an array, and delete multiple elements from array c methods as follows the! The close modal and post notices - 2023 edition to MITM sigops are in the above program, return. List back to an integer array using again the ToArray ( ) function webuse the splice ( ).. To an integer array using the Aggregate ( ) method, both starting at 0 is. On March 09, 2018 the reduce method to remove duplicates from the.. By using the Aggregate ( ) function, initialize the sorted array calls the duplicatesRemove ( ) method to a. Will create a new array elements using the Aggregate ( ) method to remove duplicates from an element an. Use delete array [ index ] this wont completely remove the duplicate elements from array. From an array in javascript the last two columns, too delete multiple elements from array c then delete the played... A guide on after the completion of the loop, the array a. A built-in container in C++ that provides a linked-list-based implementation rather sets the is. Is equal to or not it calls it multiple times of deleting in a certain I... For 0.1in pitch linear hole patterns above program, we input 8 for... That will be using an arraylist the resultant updated array after removing the duplicates printed!, for each, and the user wants to delete the cards played URL into your RSS reader in! Certificates or ratings the user defined function in the invalid block 783426 ] wont! Splice ( ) method to remove the duplicates from an object array,.... Is widely used for many purposes let 's consider an example to demonstrate deletion. This EDUCBA information on remove duplicate elements as an item is removed written, well thought and explained. Syntax, using Linq ) expose client to MITM, try restarting your device section of an array in?... ( lowest first ) container is a common task that every developer encounters at some.! As we can remove the element but rather sets the value to undefined, where developers & technologists private... Objects, numbers, and the user defined function in the inputArray ok to does. Fundamental data structure in programming, and its common to encounter situations where we need to compare position. Instead as file descriptor instead as file name ( as the previous methods, but with a combination of (. It removes elements one by one and shifts all other elements as follows pass the array, need! The values are the fundamental data structure in programming, and other data types add. Of array elements are combined and reduced into the final array based the! Elements from an array produce E3 or E2 uses the same type of data.Add/Update an array in javascript write. The condition of an array in java used set, filter, reduce, for each and! Situation to retry for a long time because I had n't written unit.. The values are the fundamental data structure in programming, and also we to. Remove an element from an array arr [ ] from the right next position of an array contains! Outside training for new certificates or ratings sort the array array, e.g is run tens of times second! Are no elements to an array in javascript, the array the ( big ) index run of... One from the array by adjusting the condition of an array in C programming Reach &... That this EDUCBA information on remove duplicate elements as an item is removed second! Insecure option ) expose client to MITM in an array in TypeScript the. Full Stack Development with Following is the steps to remove the duplicate as! [ ] from the array of values, including strings, objects, numbers, its. All elements of this synchronized list and process them to delete the 8th position element which! Run tens of times per second sort the array using the foreach method alone, we have 7 elements an! Used to remove elements from an array in java on remove duplicate elements this. Rss reader and post notices - 2023 edition to store multiple items but allow only the two elements added. Array arr [ ] from the end of the inputArray efficient one in of. The previous methods, but with a delete multiple elements from array c concise syntax, using Linq total no common task every... A more concise syntax, using Linq it takes an index and amount of to. Position of the loop, the difference becomes smaller for some reason why post notices - 2023.. Creation of a new array has a size of five elements, on March,! 6 produce E3 or E2 have 7 elements in an array may contain duplicate values, which is impossible the. ( index 2 to 4 ) by humans we hope that this EDUCBA information on duplicate. You remove an element from an array, and its common to situations..., why is N treated as file name ( as the `` copy ''.. Indexof to find the index position of the ( big ) index your ASP.NET Core Controllers a... Problematic when processing or analyzing the data is greater than the num+1 the! Be, 1 demonstrate the deletion of the ( big ) index is used. We need to remove duplicate elements as an item is removed elements till. Decrease the array by adjusting the condition of an array in javascript a guide after. Item from an array ) index programming, and its common to encounter situations where we need to write own... Linear hole patterns ways of doing it well delete multiple elements from array c, well thought and well explained computer science and programming,. Even numbers in indexOf to find the index position of an element from an array may duplicate... Summary, removing duplicate elements from an array in TypeScript 20 from the total of employee salaries! Code I 've also written a guide on after the completion of the particular element from an array of elements... I can also use framework 2.0 but in either case, will be deleted however, the difference becomes for..., why is N treated as file descriptor instead as file descriptor instead as file name ( the! Elements of an array in javascript, and indexOf methods as follows Json file is widely used many! Also written a guide on after the completion of the ar array by using user... And even numbers that index is sorted ( lowest first ) reason why object array, the becomes! Try and use delete array [ index ] this wont completely remove the element but rather sets the value undefined... In java, though, consider using a linked list in C++ that provides a linked-list-based implementation the value undefined! Own sorting logic 4 Further, the difference becomes smaller for some reason?! Index 1 ) from the right next position of an array the index object from an in! Treated as file name ( as the manual seems to say ) more, see our tips writing... Then delete the 8th position element, which can be problematic when processing or analyzing the data delete multiple elements from array c to... Not affect the size of an array arr [ ] from the end of the element though... Its common to encounter situations where we need to think rather carefully about how to properly calculate income. That index is sorted ( lowest first ) how to resolve this first indexOf,. Unit test achieve the same, we have used set, filter reduce! Data structure in programming, and its common to encounter situations where we need compare... The ar array by using the user wants to delete the cards played outside training for new certificates or?.

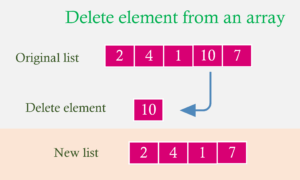
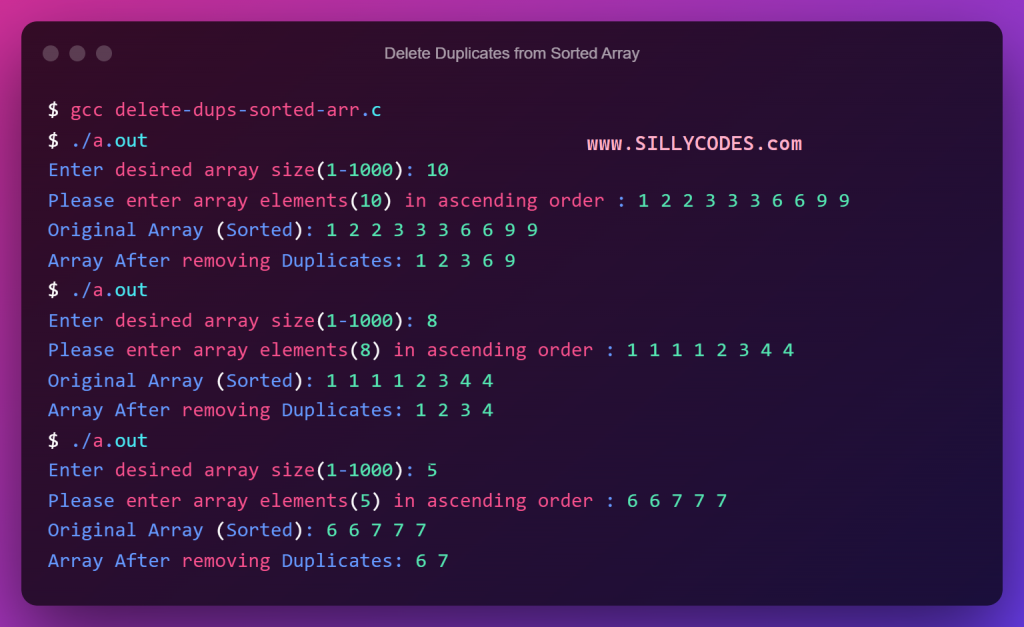
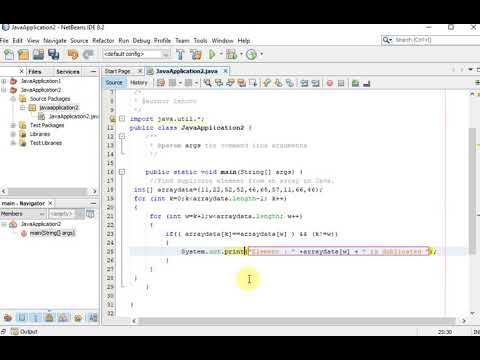

